Try out this solitaire game. This solitaire game is fun and addictive. For rules of this game, click here. It also saves games, tells you when there are no more moves,when aces are covering each piles and custom card decks. Just run the exe file to play. Now available for windows 3.1 as well, download acesup16.exe for the 16-bit version. You can now purchase the source code password. The password will work for all new versions. You can buy 32-bit, 16-bit or both source codes.
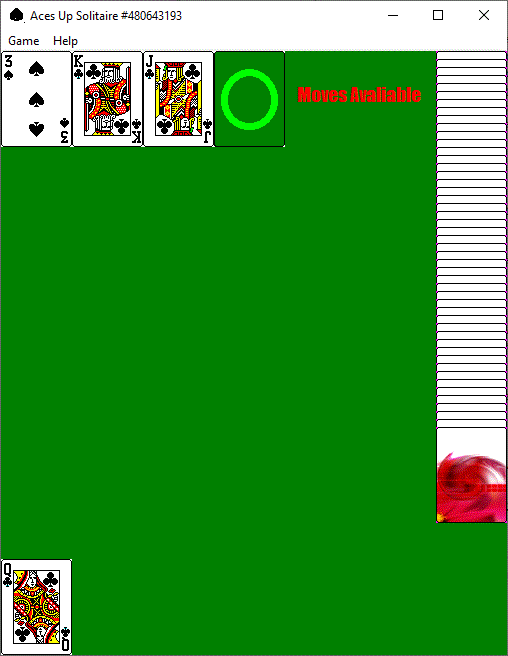