It has features such as:
* Can use UTC instead of local timezone
* Use a different TimeZone rather than what windows was setup for.
* Can display 12-hour format time(default) or 24-hour format.
* Can change the way things look in it, such as LED Colors,LED Styles, Background color and font.
NEW VERSION 3.0
* can put the clock onto wallpaper
* use the delphijustin time server or your own.
* You can now have separate LED colors.
* Have it update the time or show the pattern used in binary clock to tell you that the time needs to be set(just like a real binary clock)
* A optional AM/PM LED
New improvement in 2.0 is the design, works with control panel preview monitor and many more!
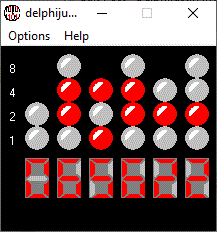
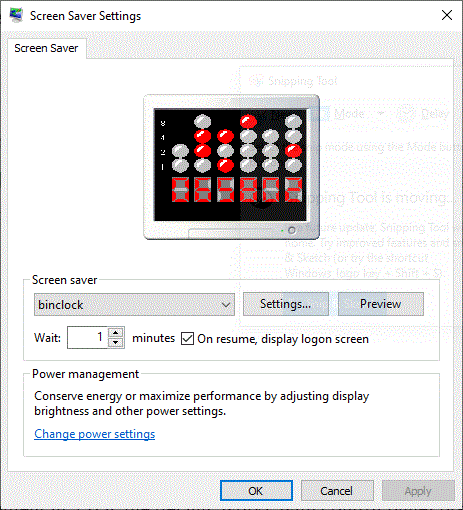